在 Visual Basic 6.0及以前版本中,都不支持對于控制臺應用程序的編程。在VB.net開始后,VB能夠支持最簡單直接的命令行編程,其用法和C、C++等非常類似。以MSDN中列舉的例子為例,可以很快速的完成控制臺方式的程序。
' A "Hello, World!" program in Visual Basic.
Module Hello
Function Main(ByVal cmdArgs() As String) As Integer
'MsgBox("Hello, World!") ' Display message on computer screen.
console.clear()
console.writeline("這是一個控制臺輸出的測試程序。下面是你輸入的命令行參數(shù):")
dim sp as string
sp=""
for each s in cmdargs
sp=sp+s+" "
next
console.writeline(sp)
console.writeline("Hello, 你輸入了" & cmdargs.length().tostring & "個變量,請按任意鍵結束程序。。。")
console.read()
Main=cmdargs.length()
End function
End Module
以上是一個完整的Console應用,把用戶在命令行的輸入顯示出來,然后顯示輸入變量的個數(shù)。等待用戶按任意鍵以后結束運行。
把以上的代碼存在一個hello.vb的文本文件中,在命令行下使用VB的編譯工具 VBC進行編譯。如果沒有出錯,則不會有任何額外顯示。如果出錯,則顯示出錯誤的內(nèi)容和位置,修改后重新編譯即可。最終生成hello.exe可執(zhí)行文件。
運行hello.exe,并且在后面附加任意的內(nèi)容,如"This is a test"作為該程序的輸入?yún)?shù),運行結果如下:
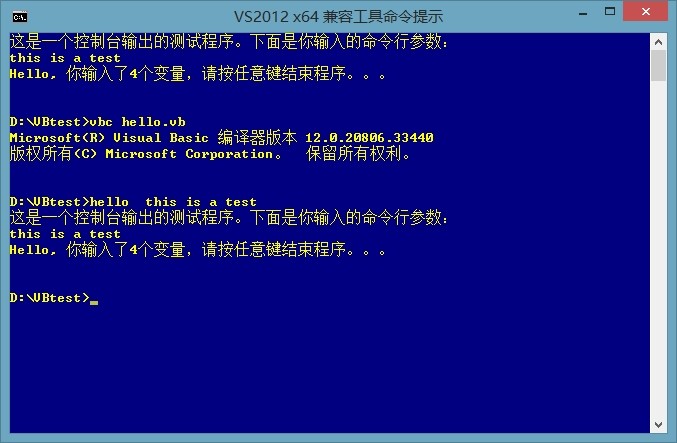
因為在程序中,選擇的是帶有返回值的Main函數(shù)形式,因此,最終把輸入變量的個數(shù)返回給系統(tǒng),可以使用 ERRORLEVEL這個環(huán)境變量來檢測其結果。示例如下:echo %errorlevel%,顯示的結果是4。
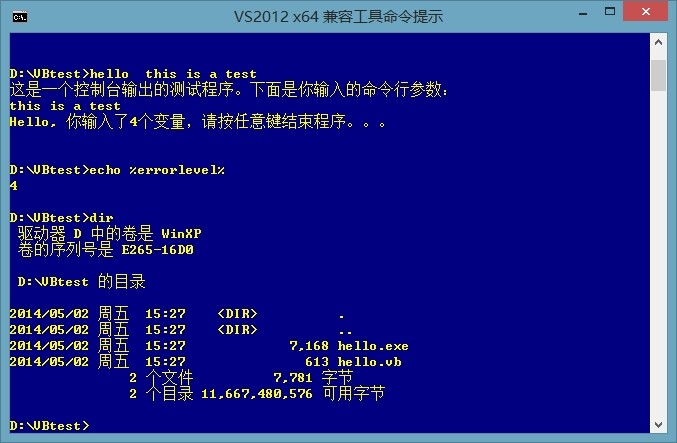
檢查這個目錄的全部文件,可以看到,源文件僅包含 hello.vb一個文件,大小613字節(jié)。編譯后的運行程序也僅有7168字節(jié),非常簡練。對于一下簡單工作,就不需要編寫復雜的界面,只要完成核心工作即可。示例如下:
Imports System
Imports System.IO
Imports System.Text
Module TypeTxt
Public txtFile As StreamReader
Public strTmp As String
''' <summary>
''' 整個程序的主函數(shù)
''' </summary>
Function Main(ByVal cmdArgs() As String) As Integer
'MsgBox("此應用程序只是演示在控制臺模式下讀取文件的例子。")
Dim returnValue As Integer = 0
Dim sp As String = ""
' See if there are any arguments.
Console.WriteLine("這次輸入了 " & cmdArgs.Length.ToString & " 個參數(shù),分別列舉如下:")
'If cmdArgs.Length > 0 Then
For argNum As Integer = 0 To UBound(cmdArgs, 1)
sp = cmdArgs(argNum)
Console.WriteLine(sp)
Next argNum
'End If
If cmdArgs.Length = 0 Then
Console.WriteLine("沒有指定文件。程序退出。")
Main = -1
Exit Function
End If
If cmdArgs.Length > 1 Then sp = cmdArgs(1)
returnValue = readtxt(sp)
' Insert call to appropriate starting place in your code.
' On return, assign appropriate value to returnValue.
' 0 usually means successful completion.
'MsgBox("The application is terminating with error level " & CStr(returnValue) & ".")
Return returnValue
End Function
''' <summary>
''' 讀文件并顯示的過程
''' </summary>
Public Function readtxt(theFile As String) As Boolean
Dim curEnc As Encoding
Dim ansi As Encoding = Encoding.Default
Dim utf8 As Encoding = Encoding.UTF8
Dim ansiBytes As Byte()
Dim utf8Bytes As Byte()
Dim nLineNo As Long = 0
txtFile = New StreamReader(theFile)
If txtFile Is Nothing Then
Console.WriteLine("打開文件錯誤,退出。")
readtxt = False
Exit Function
End If
curEnc = txtFile.CurrentEncoding
Console.WriteLine()
Console.WriteLine("當前文件編碼為:" & curEnc.ToString)
Do
strTmp = txtFile.ReadLine
‘MsgBox(strTmp)
nLineNo += 1
If strTmp Is Nothing Or nLineNo > 100 Then Exit Do
ansiBytes = ansi.GetBytes(strTmp)
utf8Bytes = Encoding.Convert(ansi, utf8, ansiBytes)
Dim uniChars(utf8.GetCharCount(utf8Bytes, 0, utf8Bytes.Length) - 1) As Char
utf8.GetChars(utf8Bytes, 0, utf8Bytes.Length, uniChars, 0)
Dim utfString As New String(uniChars)
Console.WriteLine(nLineNo.ToString() & ": " & uniChars)
Loop
txtFile.Close()
Console.WriteLine("顯示完成,按任意鍵繼續(xù)...")
Console.Read()
readtxt = True
End Function
End Module
以上是一個簡單的顯示文件內(nèi)容的例子。但是因為Windows下ANSI編碼和UTF-8編碼的問題,對于UTF-8編碼的文本,可以正確顯示其中的漢字,但是如果使用ANSI編碼格式,漢字則被顯示為一連串的???。這個問題還沒找到答案。
|