|
#include <stdio.h>
#include <conio.h>
#include <stdlib.h>
int Year=2012;
int Month=4;
int MonthDays[] = {31 , 28 , 31 , 30 , 31 , 30 , 31 , 31 , 30 , 31 , 30 , 31};
char IsLeapYear(int Year)
{
if((Year % 4 == 0 && Year % 100 != 0) || Year % 400 == 0)
{
return 1 ;
}
else
{
return 0 ;
}
}
int GetFirstDay(int year,int month,int day)
{
int week=0;
int c=0,y=0;
if (month==1)
{
year--;
month=13;
}
if (month==2)
{
year--;
month=14;
}
c=year/100;
y=year%100;
week=(c/4)-2*c+y+(y/4)+(26*(month+1)/10)+day-1;
week%=7;
if (week<0)
{
week+=7;
}
return week;
}
void DisMonthDays(int year,int month)
{
int i;
int tmp=0;
int week=0;
printf(" %d 年 %d 月\n",year,month);
printf("日 一 二 三 四 五 六\n");
tmp=MonthDays[month-1];
if (month==2)
{
if (IsLeapYear(year))
{
tmp=MonthDays[month-1]+1;
}
else
tmp=MonthDays[month-1];
}
week=GetFirstDay(year,month,1);
for(i=0;i<week;i++)
{
printf(" ");
}
for(i=1;i<=tmp;i++)
{
printf("%2d ",i);
if (week>=6)
{
week=0;
printf("\n");
}
else
week++;
}
}
void GetKey()
{
int key;
key = getche();
if(key==224)
{
key = getche();
if (key==72)
{
Month++;
if (Month>12)
{
Month=1;
Year++;
}
}
else
if (key==80)
{
Month--;
if (Month<1)
{
Month=12;
Year--;
}
}
}
}
void main(void)
{
while (1)
{
DisMonthDays(Year,Month);
GetKey();
printf("\n");
system("cls");
}
}
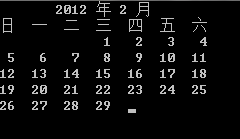
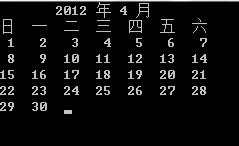
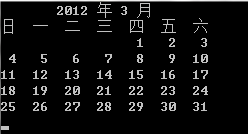
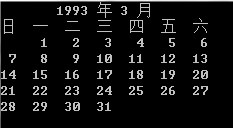
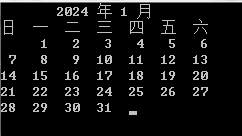
|
|