App Face
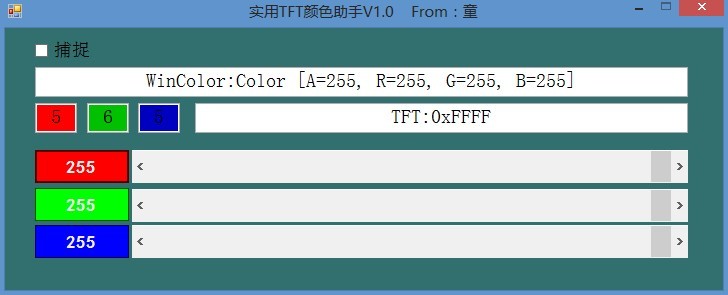
/*****Form1 Source Code ****************************************/
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace 取顏色
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private int R=255, G=255, B=255;
private int RU = 5, GU = 6, BU = 5;
private void Form1_Load(object sender, EventArgs e)
{
SetColorToButton();
}
private void button2_Click(object sender, EventArgs e)
{ }
private void hScrollBar1_ValueChanged(object sender, EventArgs e)
{
SetColorToButton();
}
private void hScrollBar2_ValueChanged(object sender, EventArgs e)
{
SetColorToButton();
}
private void hScrollBar3_ValueChanged(object sender, EventArgs e)
{
SetColorToButton();
}
private void SetColorToButton()
{
int C;
RU = int.Parse(textBox3.Text.Trim());
GU = int.Parse(textBox4.Text.Trim());
BU = int.Parse(textBox5.Text.Trim());
R = hScrollBar1.Value;
G = hScrollBar2.Value;
B = hScrollBar3.Value;
button1.Text = R.ToString();
button8.Text = G.ToString();
button2.Text = B.ToString();
button2.BackColor = Color.FromArgb(0, 0, B);
button8.BackColor = Color.FromArgb(0, G, 0);
button1.BackColor = Color.FromArgb(R, 0, 0);
textBox1.BackColor = Color.FromArgb(R, G, B);
textBox2.BackColor = Color.FromArgb(R, G, B);
textBox1.ForeColor= Color.FromArgb(255-R, 255-G, 255-B);
textBox2.ForeColor = Color.FromArgb(255 - R, 255 - G, 255 - B);
textBox1.Text = "WinColor:" + Color.FromArgb(R, G, B).ToString();
C = 0;
C = (R * (int)Math.Pow(2, RU) / 256); C <<= GU;
C += (G * (int)Math.Pow(2, GU) / 256); C <<= BU;
C+= (B*(int)Math.Pow(2, BU)/256);
textBox2.Text = "TFT:0x" + C.ToString("X");
}
private void timer1_Tick(object sender, EventArgs e)
{
if (checkBox1.Checked == true)
{
textBox1.BackColor = ColorPickerManager.GetColor(MousePosition.X, MousePosition.Y);
hScrollBar1.Value = textBox1.BackColor.R;
hScrollBar2.Value= textBox1.BackColor.G;
hScrollBar3.Value=textBox1.BackColor.B;
SetColorToButton();
}
}
}
}
/**************************Form1 End*********************************************************************/
/*************************GetColor Class******************************************************************/
using System;
using System.Drawing;
using System.Runtime.InteropServices;
namespace 取顏色
{
/// <summary>
/// 獲取當前光標處顏色,win8下wpf測試成功
/// </summary>
public static class ColorPickerManager
{
/// <summary>
///
/// </summary>
/// <param >鼠標相對于顯示器的坐標X</param>
/// <param >鼠標相對于顯示器的坐標Y</param>
/// <returns></returns>
public static Color GetColor(int x, int y)
{
IntPtr hdc = GetDC(IntPtr.Zero);
uint pixel = GetPixel(hdc, x, y);
ReleaseDC(IntPtr.Zero, hdc);
Color color = Color.FromArgb((int)(pixel & 0x000000FF), (int)(pixel & 0x0000FF00) >> 8, (int)(pixel & 0x00FF0000) >> 16);
return color;
}
[DllImport("gdi32.dll")]
private static extern bool BitBlt(
IntPtr hdcDest, // 目標設備的句柄
int nXDest, // 目標對象的左上角的X坐標
int nYDest, // 目標對象的左上角的X坐標
int nWidth, // 目標對象的矩形的寬度
int nHeight, // 目標對象的矩形的長度
IntPtr hdcSrc, // 源設備的句柄
int nXSrc, // 源對象的左上角的X坐標
int nYSrc, // 源對象的左上角的X坐標
int dwRop // 光柵的操作值
);
[DllImport("gdi32.dll")]
private static extern IntPtr CreateDC(
string lpszDriver, // 驅動名稱
string lpszDevice, // 設備名稱
string lpszOutput, // 無用,可以設定位"NULL"
IntPtr lpInitData // 任意的打印機數據
);
/// <summary>
/// 獲取指定窗口的設備場景
/// </summary>
/// <param >將獲取其設備場景的窗口的句柄。若為0,則要獲取整個屏幕的DC</param>
/// <returns>指定窗口的設備場景句柄,出錯則為0</returns>
[DllImport("user32.dll")]
private static extern IntPtr GetDC(IntPtr hwnd);
/// <summary>
/// 釋放由調用GetDC函數獲取的指定設備場景
/// </summary>
/// <param >要釋放的設備場景相關的窗口句柄</param>
/// <param >要釋放的設備場景句柄</param>
/// <returns>執行成功為1,否則為0</returns>
[DllImport("user32.dll")]
private static extern Int32 ReleaseDC(IntPtr hwnd, IntPtr hdc);
/// <summary>
/// 在指定的設備場景中取得一個像素的RGB值
/// </summary>
/// <param >一個設備場景的句柄</param>
/// <param >邏輯坐標中要檢查的橫坐標</param>
/// <param >邏輯坐標中要檢查的縱坐標</param>
/// <returns>指定點的顏色</returns>
[DllImport("gdi32.dll")]
private static extern uint GetPixel(IntPtr hdc, int nXPos, int nYPos);
}
}
/************************************End Get Color Class***********************************************************************/
/************************************Form1 Designer Source Code*************************************************************/
namespace 取顏色
{
partial class Form1
{
/// <summary>
/// 必需的設計器變量。
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// 清理所有正在使用的資源。
/// </summary>
/// <param >如果應釋放托管資源,為 true;否則為 false。</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows 窗體設計器生成的代碼
/// <summary>
/// 設計器支持所需的方法 - 不要
/// 使用代碼編輯器修改此方法的內容。
/// </summary>
private void InitializeComponent()
{
this.components = new System.ComponentModel.Container();
this.button1 = new System.Windows.Forms.Button();
this.hScrollBar1 = new System.Windows.Forms.HScrollBar();
this.hScrollBar2 = new System.Windows.Forms.HScrollBar();
this.button8 = new System.Windows.Forms.Button();
this.hScrollBar3 = new System.Windows.Forms.HScrollBar();
this.button2 = new System.Windows.Forms.Button();
this.textBox1 = new System.Windows.Forms.TextBox();
this.textBox2 = new System.Windows.Forms.TextBox();
this.textBox3 = new System.Windows.Forms.TextBox();
this.textBox4 = new System.Windows.Forms.TextBox();
this.textBox5 = new System.Windows.Forms.TextBox();
this.checkBox1 = new System.Windows.Forms.CheckBox();
this.timer1 = new System.Windows.Forms.Timer(this.components);
this.SuspendLayout();
//
// button1
//
this.button1.BackColor = System.Drawing.Color.Red;
this.button1.FlatStyle = System.Windows.Forms.FlatStyle.Popup;
this.button1.Font = new System.Drawing.Font("楷體", 12.75F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((byte)(134)));
this.button1.ForeColor = System.Drawing.SystemColors.ButtonFace;
this.button1.Location = new System.Drawing.Point(30, 122);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(94, 33);
this.button1.TabIndex = 0;
this.button1.Text = "255";
this.button1.UseVisualStyleBackColor = false;
//
// hScrollBar1
//
this.hScrollBar1.Location = new System.Drawing.Point(127, 122);
this.hScrollBar1.Maximum = 264;
this.hScrollBar1.Name = "hScrollBar1";
this.hScrollBar1.Size = new System.Drawing.Size(556, 33);
this.hScrollBar1.TabIndex = 6;
this.hScrollBar1.Value = 255;
this.hScrollBar1.ValueChanged += new System.EventHandler(this.hScrollBar1_ValueChanged);
//
// hScrollBar2
//
this.hScrollBar2.Location = new System.Drawing.Point(127, 161);
this.hScrollBar2.Maximum = 264;
this.hScrollBar2.Name = "hScrollBar2";
this.hScrollBar2.Size = new System.Drawing.Size(556, 33);
this.hScrollBar2.TabIndex = 8;
this.hScrollBar2.Value = 255;
this.hScrollBar2.ValueChanged += new System.EventHandler(this.hScrollBar2_ValueChanged);
//
// button8
//
this.button8.BackColor = System.Drawing.Color.FromArgb(((int)(((byte)(0)))), ((int)(((byte)(192)))), ((int)(((byte)(0)))));
this.button8.FlatStyle = System.Windows.Forms.FlatStyle.Popup;
this.button8.Font = new System.Drawing.Font("楷體", 12.75F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((byte)(134)));
this.button8.ForeColor = System.Drawing.SystemColors.ButtonFace;
this.button8.Location = new System.Drawing.Point(30, 160);
this.button8.Name = "button8";
this.button8.Size = new System.Drawing.Size(94, 33);
this.button8.TabIndex = 7;
this.button8.Text = "255";
this.button8.UseVisualStyleBackColor = false;
//
// hScrollBar3
//
this.hScrollBar3.Location = new System.Drawing.Point(127, 197);
this.hScrollBar3.Maximum = 264;
this.hScrollBar3.Name = "hScrollBar3";
this.hScrollBar3.Size = new System.Drawing.Size(556, 33);
this.hScrollBar3.TabIndex = 10;
this.hScrollBar3.Value = 255;
this.hScrollBar3.ValueChanged += new System.EventHandler(this.hScrollBar3_ValueChanged);
//
// button2
//
this.button2.BackColor = System.Drawing.Color.FromArgb(((int)(((byte)(0)))), ((int)(((byte)(0)))), ((int)(((byte)(192)))));
this.button2.FlatStyle = System.Windows.Forms.FlatStyle.Popup;
this.button2.Font = new System.Drawing.Font("楷體", 12.75F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((byte)(134)));
this.button2.ForeColor = System.Drawing.SystemColors.ButtonFace;
this.button2.Location = new System.Drawing.Point(30, 197);
this.button2.Name = "button2";
this.button2.Size = new System.Drawing.Size(94, 33);
this.button2.TabIndex = 9;
this.button2.Text = "255";
this.button2.UseVisualStyleBackColor = false;
//
// textBox1
//
this.textBox1.Font = new System.Drawing.Font("宋體", 15F);
this.textBox1.Location = new System.Drawing.Point(30, 39);
this.textBox1.Name = "textBox1";
this.textBox1.Size = new System.Drawing.Size(653, 30);
this.textBox1.TabIndex = 11;
this.textBox1.TextAlign = System.Windows.Forms.HorizontalAlignment.Center;
//
// textBox2
//
this.textBox2.Font = new System.Drawing.Font("宋體", 15F);
this.textBox2.Location = new System.Drawing.Point(190, 75);
this.textBox2.Name = "textBox2";
this.textBox2.Size = new System.Drawing.Size(493, 30);
this.textBox2.TabIndex = 12;
this.textBox2.TextAlign = System.Windows.Forms.HorizontalAlignment.Center;
//
// textBox3
//
this.textBox3.BackColor = System.Drawing.Color.Red;
this.textBox3.Font = new System.Drawing.Font("宋體", 15F);
this.textBox3.ForeColor = System.Drawing.Color.Black;
this.textBox3.Location = new System.Drawing.Point(30, 75);
this.textBox3.Name = "textBox3";
this.textBox3.Size = new System.Drawing.Size(42, 30);
this.textBox3.TabIndex = 13;
this.textBox3.Text = "5";
this.textBox3.TextAlign = System.Windows.Forms.HorizontalAlignment.Center;
//
// textBox4
//
this.textBox4.BackColor = System.Drawing.Color.FromArgb(((int)(((byte)(0)))), ((int)(((byte)(192)))), ((int)(((byte)(0)))));
this.textBox4.Font = new System.Drawing.Font("宋體", 15F);
this.textBox4.Location = new System.Drawing.Point(82, 75);
this.textBox4.Name = "textBox4";
this.textBox4.Size = new System.Drawing.Size(42, 30);
this.textBox4.TabIndex = 14;
this.textBox4.Text = "6";
this.textBox4.TextAlign = System.Windows.Forms.HorizontalAlignment.Center;
//
// textBox5
//
this.textBox5.BackColor = System.Drawing.Color.FromArgb(((int)(((byte)(0)))), ((int)(((byte)(0)))), ((int)(((byte)(192)))));
this.textBox5.Font = new System.Drawing.Font("宋體", 15F);
this.textBox5.Location = new System.Drawing.Point(133, 75);
this.textBox5.Name = "textBox5";
this.textBox5.Size = new System.Drawing.Size(42, 30);
this.textBox5.TabIndex = 15;
this.textBox5.Text = "5";
this.textBox5.TextAlign = System.Windows.Forms.HorizontalAlignment.Center;
//
// checkBox1
//
this.checkBox1.AutoSize = true;
this.checkBox1.Font = new System.Drawing.Font("宋體", 13F);
this.checkBox1.ForeColor = System.Drawing.Color.Black;
this.checkBox1.Location = new System.Drawing.Point(30, 12);
this.checkBox1.Name = "checkBox1";
this.checkBox1.Size = new System.Drawing.Size(63, 22);
this.checkBox1.TabIndex = 16;
this.checkBox1.Text = "捕捉";
this.checkBox1.UseVisualStyleBackColor = true;
//
// timer1
//
this.timer1.Enabled = true;
this.timer1.Interval = 10;
this.timer1.Tick += new System.EventHandler(this.timer1_Tick);
//
// Form1
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 12F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.AutoSizeMode = System.Windows.Forms.AutoSizeMode.GrowAndShrink;
this.BackColor = System.Drawing.Color.FromArgb(((int)(((byte)(49)))), ((int)(((byte)(112)))), ((int)(((byte)(111)))));
this.ClientSize = new System.Drawing.Size(718, 262);
this.Controls.Add(this.checkBox1);
this.Controls.Add(this.textBox5);
this.Controls.Add(this.textBox4);
this.Controls.Add(this.textBox3);
this.Controls.Add(this.textBox2);
this.Controls.Add(this.textBox1);
this.Controls.Add(this.hScrollBar3);
this.Controls.Add(this.button2);
this.Controls.Add(this.hScrollBar2);
this.Controls.Add(this.button8);
this.Controls.Add(this.hScrollBar1);
this.Controls.Add(this.button1);
this.FormBorderStyle = System.Windows.Forms.FormBorderStyle.Fixed3D;
this.MaximizeBox = false;
this.Name = "Form1";
this.StartPosition = System.Windows.Forms.FormStartPosition.CenterScreen;
this.Text = "實用TFT顏色助手V1.0 From:童";
this.Load += new System.EventHandler(this.Form1_Load);
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.Button button1;
private System.Windows.Forms.HScrollBar hScrollBar1;
private System.Windows.Forms.HScrollBar hScrollBar2;
private System.Windows.Forms.Button button8;
private System.Windows.Forms.HScrollBar hScrollBar3;
private System.Windows.Forms.Button button2;
private System.Windows.Forms.TextBox textBox1;
private System.Windows.Forms.TextBox textBox2;
private System.Windows.Forms.TextBox textBox3;
private System.Windows.Forms.TextBox textBox4;
private System.Windows.Forms.TextBox textBox5;
private System.Windows.Forms.CheckBox checkBox1;
private System.Windows.Forms.Timer timer1;
}
}
/************************************End Form1 Designer Source Code*************************************************************/
|