|
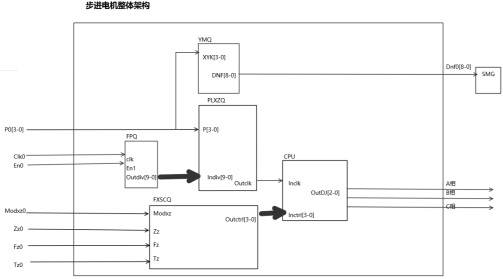 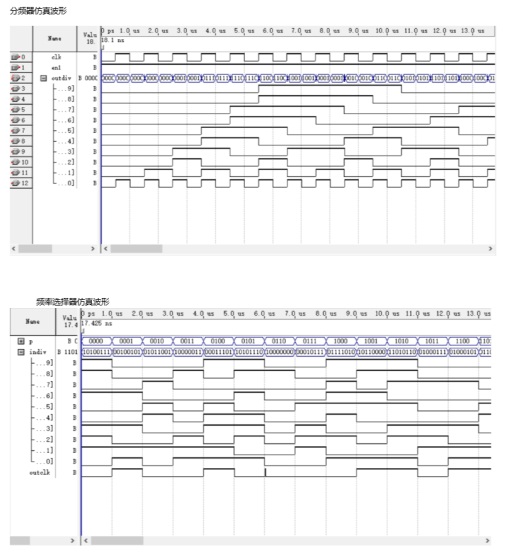 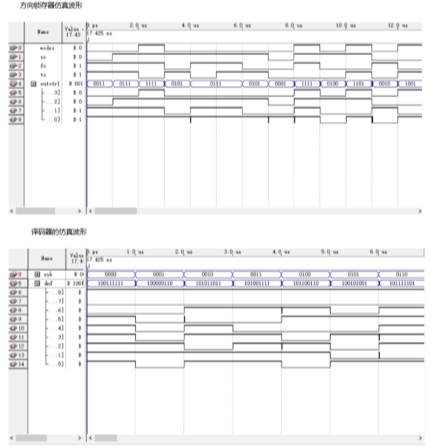 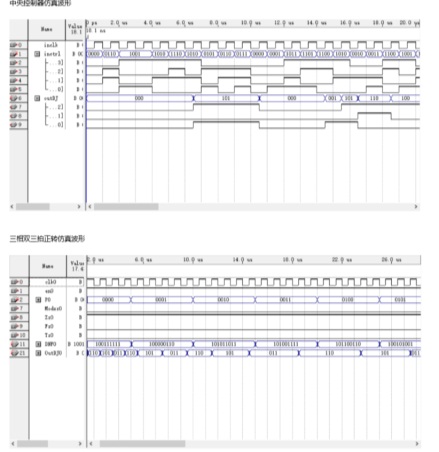 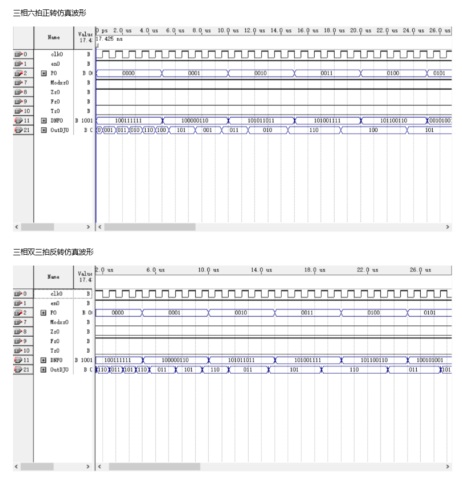 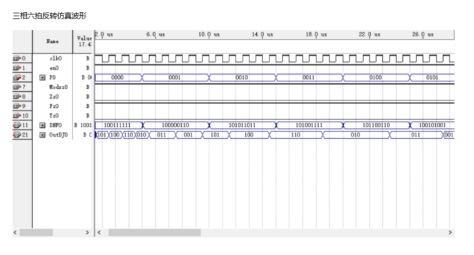 - //******模塊一:分頻器*****************//
- /*en1為使能端,clk為輸入頻率,outdiv(0-9)為輸出頻率,
- outdiv(0)為不進行分頻,outdiv(1)進行2分頻,
- outdiv(2)進行3分頻,outdiv(3)進行4分頻,
- outdiv(4)進行5分頻,outdiv(5)進行6分頻,
- outdiv(6)進行7分頻,outdiv(7)進行8分頻,
- outdiv(8)進行9分頻,outdiv(9)進行10分頻。*/
- library ieee;
- use ieee.std_logic_1164.all;
- entity FPQ is
- port(clk,en1: in std_logic;
- outdiv : out std_logic_vector(9 downto 0));
- end FPQ;
- architecture bh of FPQ is
- begin
- process(en1,clk)
- variable t1 : integer range 0 to 2;
- variable t2 : integer range 0 to 3;
- variable t3 : integer range 0 to 4;
- variable t4 : integer range 0 to 5;
- variable t5 : integer range 0 to 6;
- variable t6 : integer range 0 to 7;
- variable t7 : integer range 0 to 8;
- variable t8 : integer range 0 to 9;
- variable t9 : integer range 0 to 10;
- begin
- outdiv(0) <= clk;
- if clk'event and clk = '1' then
- if en1 = '1' then
- if (t1 >= 2) then t1:=0;outdiv(1)<='0';
- elsif t1<1 then outdiv(1)<='0';
- else outdiv(1) <= '1';
- end if;
- t1:=t1+1;
- if (t2 >= 3) then t2:=0;outdiv(2)<='0';
- elsif t2<2 then outdiv(2)<='0';
- else outdiv(2) <= '1';
- end if;
- t2:=t2+1;
- if (t3 >= 4) then t3:=0;outdiv(3)<='0';
- elsif t3<2 then outdiv(3)<='0';
- else outdiv(3) <= '1';
- end if;
- t3:=t3+1;
- if (t4 >= 5) then t4:=0;outdiv(4)<='0';
- elsif t4<3 then outdiv(4)<='0';
- else outdiv(4) <= '1';
- end if;
- t4:=t4+1;
- if (t5 >= 6) then t5:=0;outdiv(5)<='0';
- elsif t5<3 then outdiv(5)<='0';
- else outdiv(5) <= '1';
- end if;
- t5:=t5+1;
- if (t6 >= 7) then t6:=0;outdiv(6)<='0';
- elsif t6<4 then outdiv(6)<='0';
- else outdiv(6) <= '1';
- end if;
- t6:=t6+1;
- if (t7 >= 8) then t7:=0;outdiv(7)<='0';
- elsif t7<4 then outdiv(7)<='0';
- else outdiv(7) <= '1';
- end if;
- t7:=t7+1;
- if (t8 >= 9) then t8:=0;outdiv(8)<='0';
- elsif t8<5 then outdiv(8)<='0';
- else outdiv(8) <= '1';
- end if;
- t8:=t8+1;
- if (t9 >= 10) then t9:=0;outdiv(9)<='0';
- elsif t9<5 then outdiv(9)<='0';
- else outdiv(9) <= '1';
- end if;
- t9:=t9+1;
- end if;
- end if;
- end process;
- end bh;
-
-
-
-
-
-
-
-
-
-
- //***********模塊二:頻率選擇器**************//
- /*P0、P1、P2、P3為頻率選擇信號,indiv(0-9)為分頻器分頻之后的頻率信號,
- outclk為要選擇的頻率。
- 當p(3-0)為"0000"時,outclk為indiv(0);當p(3-0)為"0001"時,outclk為indiv(1);
- 當p(3-0)為"0010"時,outclk為indiv(2);當p(3-0)為"0011"時,outclk為indiv(3);
- 當p(3-0)為"0100"時,outclk為indiv(4);當p(3-0)為"0101"時,outclk為indiv(5);
- 當p(3-0)為"0110"時,outclk為indiv(6);當p(3-0)為"0111"時,outclk為indiv(7);
- 當p(3-0)為"1000"時,outclk為indiv(8);當p(3-0)為"1001"時,outclk為indiv(9);
- 當p(3-0)為其它時,outclk為indiv(0)。*/
- library ieee;
- use ieee.std_logic_1164.all;
- entity PLXZQ is
- port(p: in std_logic_vector(3 downto 0);
- indiv : in std_logic_vector(9 downto 0);
- outclk : out std_logic);
- end PLXZQ;
- architecture bh1 of PLXZQ is
- begin
- process(p,indiv)
- begin
- case p is
- when "0000" => outclk <= indiv(0);
- when "0001" => outclk <= indiv(1);
- when "0010" => outclk <= indiv(2);
- when "0011" => outclk <= indiv(3);
- when "0100" => outclk <= indiv(4);
- when "0101" => outclk <= indiv(5);
- when "0110" => outclk <= indiv(6);
- when "0111" => outclk <= indiv(7);
- when "1000" => outclk <= indiv(8);
- when "1001" => outclk <= indiv(9);
- when others => outclk <= indiv(0);
- end case;
- end process;
- end bh1;
-
-
-
-
-
-
-
-
-
-
-
- //***********模塊三:方向鎖存器**************//
- /*Zz、Fz、Tz為方向和開關信號,Modxz為六拍和三拍選擇切換信號,
- outctrl為輸出的控制信號。
- 當Modxz為1時,為六拍;當Modxz為0時,為雙三拍。
- 當Tz為1時,為停止電機,當Tz為0時,為啟動電機。
- 當Zz為1、Fz為0時,為電機正轉,
- 當Zz為0、Fz為1時,為電機反轉,
- 當Zz為1、Fz為1時,為電機停止,
- 當Zz為0、Fz為0時,為電機停止。
- outctrl(0)對應Tz;outctrl(1)對應Fz;
- outctrl(2)對應Zz;outctrl(3)對應Modxz。*/
- library ieee;
- use ieee.std_logic_1164.all;
- entity FXSCQ is
- port(modxz,zz,fz,tz: in std_logic;
- outctrl: out std_logic_vector(3 downto 0));
- end FXSCQ;
- architecture bh2 of FXSCQ is
- begin
- process(modxz,zz,fz,tz)
- begin
- outctrl(0) <= tz;
- outctrl(1) <= fz;
- outctrl(2) <= zz;
- outctrl(3) <= modxz;
- if zz = '1' and fz = '1' then
- outctrl(0) <= '1';
- elsif zz = '0' and fz = '0' then
- outctrl(0) <= '1';
- end if;
- end process;
- end bh2;
-
-
-
-
-
-
- //***********模塊四:中央控制器**************//
- /*inclk為經過頻率選擇器選擇之后的輸入頻率,
- inctrl為經過方向鎖存器之后的輸入控制信號,
- outDj為三相電機的的三相電壓,
- 當inctrl為“0100”時,電機為雙三相正轉;
- 當inctrl為“0010”時,電機為雙三相反轉;
- 當inctrl為“1100”時,電機為單六相正轉;
- 當inctrl為“1010”時,電機為單六相正轉;
- 當inctrl為“xxx1”時,電機停止。*/
- library ieee;
- use ieee.std_logic_1164.all;
- use ieee.std_logic_unsigned.all;
- entity CPU is
- port(inclk: in std_logic;
- inctrl: in std_logic_vector(3 downto 0);
- outDJ: out std_logic_vector(2 downto 0));
- end CPU;
- architecture bh3 of CPU is
- signal Tmp : std_logic_vector(2 downto 0);
- begin
- process(inclk,inctrl)
- begin
- if inclk'event and inclk = '1' then
- if inctrl = "1100" then
- if Tmp = 6 then
- Tmp <= "001";
- else
- Tmp <= Tmp+1;
- end if;
- elsif inctrl = "1010" then
- if Tmp = 1 then
- Tmp <= "110";
- else
- Tmp <= Tmp-1;
- end if;
- elsif inctrl = "1000" then
- Tmp <= "000";
- elsif inctrl = "0100" then
- if Tmp = 6 then
- Tmp <= "010";
- else
- Tmp <= Tmp+2;
- end if;
- elsif inctrl = "0010" then
- if Tmp = 2 then
- Tmp <="110";
- else
- Tmp <= Tmp-2;
- end if;
- elsif inctrl = "0000" then
- Tmp <= "000";
- end if;
- end if;
- end process;
- with Tmp select
- outDJ <= "001" when "001",
- "011" when "010",
- "010" when "011",
- "110" when "100",
- "100" when "101",
- "101" when "110",
- "000" when others;
- end bh3;
-
-
-
- //***********模塊五:譯碼器**************//
- /*xyk(3-0)為輸入信號,輸入數值,dnf(8-0)為輸出控制數碼管信號。
- //dnf(8)為數碼管的位選信號。
- 當xyk為“0000”時,dnf輸出為“100111111”, 數碼管顯示‘0’;
- 當xyk為“0001”時,dnf輸出為“100000110”, 數碼管顯示‘1’;
- 當xyk為“0010”時,dnf輸出為“101011011”, 數碼管顯示‘2’;
- 當xyk為“0011”時,dnf輸出為“101001111”, 數碼管顯示‘3’;
- 當xyk為“0100”時,dnf輸出為“101100110”, 數碼管顯示‘4’;
- 當xyk為“0101”時,dnf輸出為“100101001”, 數碼管顯示‘5’;
- 當xyk為“0110”時,dnf輸出為“101111101”, 數碼管顯示‘6’;
- 當xyk為“0111”時,dnf輸出為“100000111”, 數碼管顯示‘7’;
- 當xyk為“1000”時,dnf輸出為“101111111”, 數碼管顯示‘8’;
- 當xyk為“1001”時,dnf輸出為“101100111”, 數碼管顯示‘9’;
- 當xyk為其它時,dnf輸出為“100111111”, 數碼管顯示‘0’。*/
- library ieee;
- use ieee.std_logic_1164.all;
- entity YMQ is
- port(xyk: in std_logic_vector(3 downto 0);
- dnf: out std_logic_vector(8 downto 0));
- end YMQ;
- architecture bh4 of YMQ is
- begin
- process(xyk)
- begin
- case xyk is
- when "0000" => dnf <= "100111111";
- when "0001" => dnf <= "100000110";
- when "0010" => dnf <= "101011011";
- when "0011" => dnf <= "101001111";
- when "0100" => dnf <= "101100110";
- when "0101" => dnf <= "100101001";
- when "0110" => dnf <= "101111101";
- when "0111" => dnf <= "100000111";
- when "1000" => dnf <= "101111111";
- when "1001" => dnf <= "101100111";
- when others => dnf <= "100111111";
- end case;
- end process;
- end bh4;
-
-
-
-
- //********總體:模塊的例化*************//
- library ieee;
- use ieee.std_logic_1164.all;
- entity zong is
- port(clk0,en0,Modxz0,Zz0,Fz0,Tz0 : in std_logic;
- P0 : in std_logic_vector(3 downto 0);
- DNF0 : out std_logic_vector(8 downto 0);
- OutDJ0 : out std_logic_vector(2 downto 0));
- end zong;
- architecture bh0 of zong is
- component FPQ
- port(clk,en1: in std_logic;
- outdiv : out std_logic_vector(9 downto 0));
- end component;
- component PLXZQ
- port(p: in std_logic_vector(3 downto 0);
- indiv : in std_logic_vector(9 downto 0);
- outclk : out std_logic);
- end component;
- component YMQ
- port(xyk: in std_logic_vector(3 downto 0);
- dnf: out std_logic_vector(8 downto 0));
- end component;
- component CPU
- port(inclk: in std_logic;
- inctrl: in std_logic_vector(3 downto 0);
- outDJ: out std_logic_vector(2 downto 0));
- end component;
- component FXSCQ
- port(modxz,zz,fz,tz: in std_logic;
- outctrl: out std_logic_vector(3 downto 0));
- end component;
- signal DX1 :std_logic;
- signal DX2 :std_logic_vector(9 downto 0);
- signal DX3 :std_logic_vector(3 downto 0);
- begin
- U0 : FPQ port map (clk0,en0,DX2);
- U1 : PLXZQ port map (p0,DX2,DX1);
- U2 : YMQ port map (p0,dnf0);
- U3 : CPU port map (DX1,DX3,OutDJ0);
- U4 : FXSCQ port map (Modxz0,Zz0,Fz0,Tz0,DX3);
- end bh0;
復制代碼
|
-
-
文檔.doc
2020-1-1 21:41 上傳
點擊文件名下載附件
下載積分: 黑幣 -5
684.99 KB, 下載次數: 27, 下載積分: 黑幣 -5
評分
-
查看全部評分
|