此程序為一個學生學籍管理系統,用戶可以在程序開始運行時選擇學生人數和課程人數,在輸入之后便可進行操作, 計算每科的總成績、平均成績,計算每個人的總成績、平均成績,按分數排序,按學號排序,通過學號搜索信息,通過姓名搜索信息,各科成績分析,列出所有人員信息 等功能。 2.功能模塊圖 - . 選擇學生人數和課程人數。
- . 計算每科的總成績、平均成績
- . 計算每個人的總成績、平均成績
- . 按分數排序
- .按學號排序
- . 通過學號搜索信息
- . 通過姓名搜索信息
- . 各科成績分析
- . 列出所有人員信息
2.詳細流程 首先定義結構體存放學生的基本屬性 //結構體的定義,表示每個學生的信息 typedef struct //定義了一個名字叫做student的結構體 { int school_number; //學號 char name[128]; //姓名 float object[128]; //科目 float total_sort; //總成績 float average_sort; //平均成績 }student; 接著定義了四個全局變量 //定義了四個全局變量 int how_many_people; //多少人 int how_many_object; //多少科 student theClass[30],temp; //結構體數組,表示多少個人,30可以換,temp交換用的一個結構體 char anything; //記錄任意鍵的變量名 接著定義十個函數分別完成各個的功能,每個參數引入的形式參數 void how_many_people_and_object(); //一共幾人幾科 void input_record_manually(); //輸入信息依次輸入學生名字以及課程科目平均成績 void Calculate_total_and_average_score_of_every_course(); //計算每科的總成績平均成績 運用兩個for循環統計每個科目的每個人的信息相加求出總和,除以人數求出平均值 void Calculate_total_and_average_score_of_every_student(); //計算每個人的總成績平均成績,由結構體直接讀出來 voidSort_in_descending_order_by_total_score_of_every_student();//按總成績排名 用冒泡排序 將第一個元素與之后的n個元素比較,如果有比第一個元素大的元素則將其置換,繼續比較,則一次比較完畢,最后一個元素是最大的,再將第二個元素與n-1個元素比較重復過程。 void Sort_in_ascending_order_by_number(); //按學號大小排名用冒泡排序 將第一個元素與之后的n個元素比較,如果有比第一個元素大的元素則將其置換,繼續比較,則一次比較完畢,最后一個元素是最大的,再將第二個元素與n-1個元素比較重復過程。 void Search_by_number();//通過學號來搜索 void Search_by_name();//通過姓名來搜索 void Statistic_analysis_for_every_course();//分析各科成績 void List_record();//列出信息 流程圖: 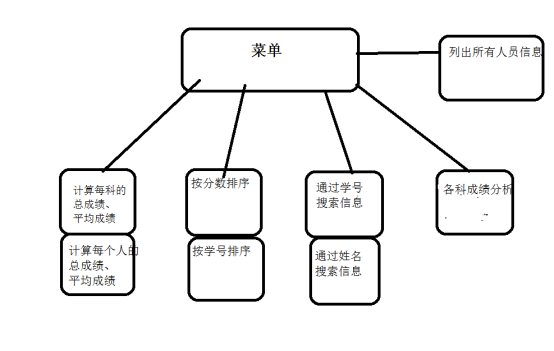
- #include<stdio.h> //C語言的頭文件
- #include<windows.h> //windows的頭文件
- #include<conio.h> //控制臺輸入輸出頭文件
- #include<time.h> //時間的頭文件
- void how_many_people_and_object(); //一共幾人幾科
- void input_record_manually(); //輸入信息
- void Calculate_total_and_average_score_of_every_course(); //計算每科的總成績平均成績
- void Calculate_total_and_average_score_of_every_student(); //計算每個人的總成績平均成績
- void Sort_in_descending_order_by_total_score_of_every_student();//按總成績排名
- void Sort_in_ascending_order_by_number(); //按學號大小排名
- void Search_by_number(); //通過學號來搜索
- void Search_by_name(); //通過姓名來搜索
- void Statistic_analysis_for_every_course(); //分析各科成績
- void List_record(); //列出信息
-
- //結構體的定義,表示每個學生的信息
- typedef struct //定義了一個名字叫做student的結構體
- {
- int school_number; //學號
- char name[128]; //姓名
- float object[128]; //科目
- float total_sort; //總成績
- float average_sort; //平均成績
- }student;
-
- //定義了四個全局變量
- int how_many_people; //多少人
- int how_many_object; //多少科
- student theClass[30],temp; //結構體數組,表示多少個人,30可以換,temp交換用的一個結構體
- char anything; //記錄任意鍵的變量名
-
- //函數的定義
- void how_many_people_and_object()
- {
- printf("請輸入學生人數:");
- scanf("%d",&how_many_people);
- printf("請輸入總科目:");
- scanf("%d",&how_many_object);
- }
- void input_record_manually()
- {
- char ch;
- for(int i=0;i<how_many_people;i++)
- {
- printf("請輸入第 %d 個人的姓名:",i+1);
- scanf("%s",&theClass[i].name);
-
- printf("請輸入第 %d 個人的學號:",i+1);
- scanf("%d",&theClass[i].school_number);
-
- float sum=0;
- for(int j=0;j<how_many_object;j++)
- {
- printf("請輸入第 %d 個人的第 %d 科成績:",i+1,j+1);
- scanf("%f",&theClass[i].object[j]);
-
- sum=sum+theClass[i].object[j];
- theClass[i].total_sort=sum;
- theClass[i].average_sort=theClass[i].total_sort/how_many_object;
- }
- printf("總成績: %.2f\n",theClass[i].total_sort);
- printf("平均成績: %.2f\n",theClass[i].average_sort);
- }
- printf("任意鍵返回主菜單");
- ch=getche();
- }
- void Calculate_total_and_average_score_of_every_course()
- {
- char ch;
- float total_sum=0;
- float average_sum=0;
- for(int j=0;j<how_many_object;j++)
- {
- for(int i=0;i<how_many_people;i++)
- {
- total_sum = theClass[i].object[j] + total_sum;
- average_sum = total_sum / how_many_people;
- }
- printf("第 %d 科的總成績:%.2f 平均成績:%.2f\n",j+1,total_sum,average_sum);
- total_sum=0;
- average_sum=0;
- }
-
- printf("任意鍵返回主菜單");
- ch=getche();
- }
- void Calculate_total_and_average_score_of_every_student()
- {
- char ch;
- for(int i=0;i<how_many_people;i++)
- {
- printf("%s 的總成績: %.2f 平均成績: %.2f\n",theClass[i].name,theClass[i].total_sort,theClass[i].average_sort);
- }
- printf("任意鍵返回主菜單");
- ch=getche();
- }
- void Sort_in_descending_order_by_total_score_of_every_student()
- {
- char ch;
- for(int i=0;i<how_many_people;i++) //冒泡排序
- {
- for(int j=i+1;j<how_many_people;j++)
- {
- if(theClass[i].total_sort<theClass[j].total_sort)
- {
- temp=theClass[i];
- theClass[i]=theClass[j];
- theClass[j]=temp;
- }
- }
- }
- for(int ii=0;ii<how_many_people;ii++)
- {
- printf("第%d名:%s--%.2f\n",ii+1,theClass[ii].name,theClass[ii].total_sort);
- }
- printf("任意鍵返回主菜單");
- ch=getche();
- }
- void Sort_in_ascending_order_by_number()
- {
- char ch;
- for(int i=0;i<how_many_people;i++)
- {
- for(int j=i+1;j<how_many_people;j++)
- {
- if(theClass[i].school_number>theClass[j].school_number)
- {
- temp=theClass[i];
- theClass[i]=theClass[j];
- theClass[j]=temp;
- }
- }
- }
- for(int ii=0;ii<how_many_people;ii++)
- {
- printf("%d--%s--%.2f\n",theClass[ii].school_number,theClass[ii].name,theClass[ii].total_sort);
- }
- printf("任意鍵返回主菜單");
- ch=getche();
- }
- void Search_by_number()
- {
- char ch;
- int number;
- printf("請輸入學號:");
- scanf("%d",&number);
- system("cls");
- for(int i=0;i<how_many_people;i++)
- {
- if(number - theClass[i].school_number == 0)
- {
- printf("姓名:%s\n",theClass[i].name);
- printf("學號:%d\n",theClass[i].school_number);
- for(int j=0;j<how_many_object;j++)
- {
- printf("第 %d 科成績:%.2f\n",j+1,theClass[i].object[j]);
- }
- printf("總成績:%.2f\n平均成績:%.2f\n\n",theClass[i].total_sort,theClass[i].average_sort);
- }
- }
- printf("任意鍵返回主菜單");
- ch=getche();
- }
- void Search_by_name()
- {
- char ch;
- char n[128];
- printf("請輸入姓名:");
- scanf("%s",&n);
- for(int i=0;i<how_many_people;i++)
- {
- if(strcmp(n,theClass[i].name)==0)
- {
- printf("姓名:%s\n",theClass[i].name);
- printf("學號:%d\n",theClass[i].school_number);
- for(int j=0;j<how_many_object;j++)
- {
- printf("第 %d科成績:%.2f\n",j+1,theClass[i].object[j]);
- }
- printf("總成績:%.2f\n平均成績:%.2f\n",theClass[i].total_sort,theClass[i].average_sort);
- }
- }
- printf("任意鍵返回主菜單");
- ch=getche();
- }
- void Statistic_analysis_for_every_course()
- {
- char ch;
- float a[6][6]={0}; //第幾科的第幾類成績(優良中差及不及),最大為6課,可以改
- for(int i=0;i<how_many_people;i++)
- {
- for(int j=0;j<how_many_object;j++)
- {
- if(theClass[i].object[j]>=90 && theClass[i].object[j]<=100)
- a[j][0]++;
- else if(theClass[i].object[j]>=80 && theClass[i].object[j]<=89)
- a[j][1]++;
- else if(theClass[i].object[j]>=70 && theClass[i].object[j]<=79)
- a[j][2]++;
- else if(theClass[i].object[j]>=60 && theClass[i].object[j]<=69)
- a[j][3]++;
- else
- a[j][4]++;
- }
- }
- for(int j=0;j<how_many_object;j++)
- {
- printf("第 %d 科優秀的人數是:%.2f 所占百分比是:%.0f %%\n",j+1,a[j][0],a[j][0]/how_many_people*100);
- printf("第 %d 科良好的人數是:%.2f 所占百分比是:%.0f %%\n",j+1,a[j][1],a[j][1]/how_many_people*100);
- printf("第 %d 科中等的人數是:%.2f 所占百分比是:%.0f %%\n",j+1,a[j][2],a[j][2]/how_many_people*100);
- printf("第 %d 科及格的人數是:%.2f 所占百分比是:%.0f %%\n",j+1,a[j][3],a[j][3]/how_many_people*100);
- printf("第 %d 科不及的人數是:%.2f 所占百分比是:%.0f %%\n\n",j+1,a[j][4],a[j][4]/how_many_people*100);
- }
- printf("任意鍵返回主菜單");
- ch=getche();
- }
- void List_record()
- {
- char ch;
- for(int i=0;i<how_many_people;i++)
- {
- printf("%s\n",theClass[i].name);
- printf("%d號\n",theClass[i].school_number);
-
- for(int j=0;j<how_many_object;j++)
- {
- printf("第 %d 科成績:%.2f\n",j+1,theClass[i].object[j]);
- }
- printf("總成績:%.2f\n平均成績:%.2f\n\n",theClass[i].total_sort,theClass[i].average_sort);
- }
- printf("任意鍵返回主菜單");
- ch=getche();
- }
-
- //主函數
- int main()
- {
- int choise;
-
-
- printf("學生成績管理系統\n\n");
- how_many_people_and_object();
-
- part:
- system("cls");
- printf("1.Input record manually\n");
- printf("2.Calculate total and average score of every course\n");
- printf("3.Calculate total and average score of every student\n");
- printf("4.Sort in descending order by total score of every student\n");
- printf("5.Sort in ascending order by number\n");
- printf("6.Search by number\n");
- printf("7.Search by name\n");
- printf("8.Statistic analysis for every course\n");
- printf("9.List record\n");
- printf("0.Exit\n");
- printf("Please input your choice:");
- scanf("%d",&choise);
- if(choise==1)
- {
- system("cls");
- input_record_manually();
- goto part;
- }
- else if(choise==2)
- {
- system("cls");
- Calculate_total_and_average_score_of_every_course();
- goto part;
- }
- else if(choise==3)
- {
- system("cls");
- Calculate_total_and_average_score_of_every_student();
- goto part;
- }
- else if(choise==4)
- {
- system("cls");
- Sort_in_descending_order_by_total_score_of_every_student();
- goto part;
- }
- else if(choise==5)
- {
- system("cls");
- Sort_in_ascending_order_by_number();
- goto part;
- }
- else if(choise==6)
- {
- system("cls");
- Search_by_number();
- goto part;
- }
- else if(choise==7)
- {
- system("cls");
- Search_by_name();
- goto part;
- }
- else if(choise==8)
- {
- system("cls");
- Statistic_analysis_for_every_course();
- goto part;
- }
- else if(choise==9)
- {
- system("cls");
- List_record();
- goto part;
- }
- else if(choise==0)
- return 0;
- else
- ;
- return 0;
- }
復制代碼
4.技術關鍵和設計心得
在這次課程設計中,我們首先對系統的整體功能進行了構思,然后用結構化分析方法進行分析,將整個系統清楚的劃分為幾個模塊,再根據每個模塊的功能編寫代碼。而且盡可能的將模塊細分,最后在進行函數的調用。我們在函數的編寫過程中,我們不僅用到了for循環、while循環和switch語句,還用到了函數之間的調用(包括遞歸調用)。由于我們是分工編寫代碼,最后需要將每個人的代碼放到一起進行調試。因為我們每個人寫的函數的思想不都一樣,所以在調試的過程中也遇到了困難,但經過我們耐心的修改,終于功夫不負有心人,我們成功了!
完整的Word格式文檔51黑下載地址:
學生學籍管理系統 - 副本.docx
(56.68 KB, 下載次數: 9)
2018-12-26 18:14 上傳
點擊文件名下載附件
|